24.11.2009
Wake On Lan ( MagicPacket ) Repeater & Sender
arduino WOL Repeater
Wake On Lan ( MagicPacket ) Repeater & Sender
Wake On Lan ( MagicPacket ) Repeater & Switcher
Wake On Lan ( MagicPacket ) Repeater & Sender
Wake On Lan ( MagicPacket ) Repeater & Switcher
Today, I just made additional function for arduino WOL Repeater.
You can select three target PCs and send MagicPacket when you push the button.
Also, It is using bjoern / arduino_osc Library for UDP.
I am using "sndmagic version 0.2" to send a magic packet from outside of network.
You can get "sndmagic" following website:
http://www.st.rim.or.jp/~yumo/pub/sndmagic_chglog.html
If you have got other software, please change the value of localPort.
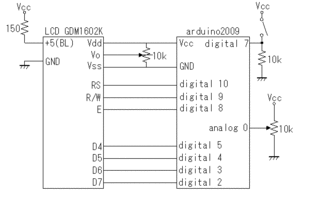
// Wake On Lan ( MagicPacket ) Repeater & Sender // http://yutakalifenet.up.seesaa.net/html/wolRepeaterSender.html // Digital 7, push button // Analog 0, variable resistor #include <WString.h> #include <Ethernet.h> #include <UdpRaw.h> #include <LiquidCrystal.h> // LiquidCrystal display with: // rs on pin 10 // rw on pin 9 // enable on pin 8 // d0, d1, d2, d3 on pins 5, 4, 3, 2 // initialize the library with the numbers of the interface pins LiquidCrystal lcd(10, 9, 8, 5, 4, 3, 2); // You can set up 3 target PCs byte TargetMac1[] = { 0x00,0x00,0x00,0x00,0x00,0x00 }; // set a MAC for hostName1 byte TargetMac2[] = { 0x00,0x00,0x00,0x00,0x00,0x00 }; // set a MAC for hostName2 byte TargetMac3[] = { 0x00,0x00,0x00,0x00,0x00,0x00 }; // set a MAC for hostName3 // 1234567890123456 please set 16 letters char hostName1[] = " hostName1 "; // set your target PC's name char hostName2[] = " hostName2 "; // set your target PC's name char hostName3[] = " hostName3 "; // set your target PC's name // ETHERNET CONFIGURATION byte mac[] = { 0xDE, 0xAD, 0xBE, 0xEF, 0xFE, 0xED }; // MAC address byte ip[] = { 192, 168, 1, 200 }; // Arduino IP address byte gw[] = { 192, 168, 1, 1 }; // Gateway IP address int localPort = 7; // local port to listen on // I am using "sndmagic version 0.2" to send a magic packet from outside of network. // You can get it following website: // http:www.st.rim.or.jp/~yumo/pub/sndmagic_chglog.html // If you have got other software, please change the value of localPort. // set a target broadcast address byte targetIp[] = { 192, 168, 1, 255}; // set this value like "x.x.x.255" int targetPort = 8000; // target port #define MAX_SIZE 192 // maximum packet size byte packetBuffer[MAX_SIZE]; // buffer to hold incoming packet int packetSize; // holds received packet size byte remoteIp[4]; // holds recvieved packet's originating IP unsigned int remotePort; // holds received packet's originating port int i; int packetLen = 102; byte packet[102]; void setup() { Ethernet.begin(mac,ip,gw); UdpRaw.begin(localPort); lcd.begin(2,16); lcd.clear(); lcd.setCursor(4,0); lcd.print("arduino"); lcd.setCursor(2,1); lcd.print("WOL Repeater"); delay(2000); lcd.clear(); // initialize D7 pin as an output: pinMode(7,INPUT); Serial.begin(9600); } void loop() { // read the value from the variable resistor and the value divide by 341: int val = analogRead(0)/341; switch (val) { case 1: lcd.print(" Target host: "); lcd.setCursor(0,1); lcd.print(hostName1); break; case 2: lcd.print(" Target host: "); lcd.setCursor(0,1); lcd.print(hostName2); break; case 3: lcd.print(" Target host: "); lcd.setCursor(0,1); lcd.print(hostName3); break; default: lcd.print(" Target host:"); lcd.setCursor(0,1); lcd.print(hostName1); } // to make a packet, if D7 input is HIGH: if(digitalRead(7)==HIGH){ delay(100); packetSize = 126; Serial.println(""); Serial.println("Sent packet to:"); Serial.println(""); for (int i = 0; i <= 5; i++){ packet[i] = 0xFF; } for (int i = 6; i <= packetLen; i++){ int j = abs((packetLen - i) % 6 - 6); if ( j == 6 ) j = 0; // to switch over by the value from the variable resistor switch (val) { case 1: packet[i] = TargetMac1[j]; break; case 2: packet[i] = TargetMac2[j]; break; case 3: packet[i] = TargetMac3[j]; break; default: packet[i] = TargetMac1[j]; } } if ( val == 1 ){ UdpRaw.sendPacket(packet,packetLen,targetIp,targetPort); lcd.clear(); lcd.print("SentMagicPacket:"); lcd.setCursor(0,1); lcd.print(hostName1); Serial.println(""); Serial.println(hostName1); Serial.println(""); delay(1000); } if ( val == 2 ){ UdpRaw.sendPacket(packet,packetLen,targetIp,targetPort); lcd.clear(); lcd.print("SentMagicPacket:"); lcd.setCursor(0,1); lcd.print(hostName2); Serial.println(""); Serial.println(hostName2); Serial.println(""); delay(1000); } if ( val == 3 ){ UdpRaw.sendPacket(packet,packetLen,targetIp,targetPort); lcd.clear(); lcd.print("SentMagicPacket:"); lcd.setCursor(0,1); lcd.print(hostName3); Serial.println(""); Serial.println(hostName3); Serial.println(""); delay(1000); } lcd.clear(); Serial.println("Magic packet ordering Done!"); for (int i = 0; i < packetLen; i++){ Serial.print(packet[i], HEX); Serial.print(" "); } Serial.println(""); Serial.println(""); } // to forward a packet, if incoming packet is available if(UdpRaw.available()) { packetSize = UdpRaw.readPacket(packetBuffer,MAX_SIZE,remoteIp,(uint16_t *)&remotePort); Serial.print("Received packet of size "); Serial.println(abs(packetSize)); Serial.print("From IP "); for(i=0; i<3; i++) { Serial.print(remoteIp[i],DEC); Serial.print("."); } Serial.print(remoteIp[3],DEC); Serial.print(" Port "); Serial.println(remotePort); if(packetSize < 0) { Serial.print("ERROR: Packet was truncated from "); Serial.print(packetSize*-1); Serial.print(" to "); Serial.print(MAX_SIZE); Serial.println(" bytes."); } Serial.println("Contents:"); for(i=0; i<min(MAX_SIZE,abs(packetSize)); i++) { Serial.print(packetBuffer[i],HEX); Serial.print(" "); } Serial.println(""); // send a magic packet UdpRaw.sendPacket(packetBuffer,packetSize,targetIp,targetPort); Serial.println("Start port forwarding to broadcast address:"); for(i=0; i<3; i++) { Serial.print(targetIp[i],DEC); Serial.print("."); } Serial.print(targetIp[3],DEC); Serial.println(""); Serial.println("Done!"); lcd.clear(); lcd.print("Received packet"); delay(2000); lcd.clear(); lcd.setCursor(4,0); lcd.print("From IP:"); lcd.setCursor(0,1); for(i=0; i<3; i++) { lcd.print(remoteIp[i],DEC); lcd.print("."); } lcd.print(remoteIp[3],DEC); delay(2000); lcd.clear(); lcd.print("Target MAC:"); lcd.setCursor(0,1); for(i=6; i<11; i++) { lcd.print(packetBuffer[i],HEX); lcd.print(":"); } lcd.print(packetBuffer[11],HEX); delay(2000); lcd.clear(); lcd.print(" Target host: "); } }